React Tutorial for Beginners
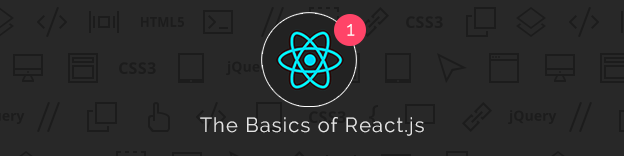
Reference link: https://ihatetomatoes.net/react-tutorial-for-beginners/
This guide is for beginner React developers who struggle to move forward while learning React.
It will give you a very simple “ABC” path that you can follow, step by step, concept by concept, without the overwhelming feeling that you need to learn everything at once.
Take your time, digest the content of each article in this series and try to complete the challenge.
My suggestion is that you only progress to the next step, when you are super clear about each of the concepts.
What you will learn
- what is a React
component
- what is the difference between
stateless
andclass
components - what is the
state
and what areprops
- what is
destructuring
in JavaScript - how to render React component on the page
Are you already familiar with React? Try the challenge at the end of the article.
1. Think React, think components
This is the first and a major concept to understand, but it’s fairly straight forward.
Every React app is build out of components. You may have one single component in a simple app or a number of components in something more complex.
Simple React components
Simple React components:
- can just render a static (hardcoded) html markup
- can be dynamically rendered based on a locally saved JSON data
- can be nested into each other to create a more complex app layout
- can be rendered based on a data (aka
props
) provided by a parent component (more on props later)
The simplest React component just renders a bit of HTML markup.
Complex React components
Given that this is a beginners guide I won’t go to much into details here.
A more complex React components might have more logic inside of them, but at the end of the day they are also just rendering a bit of HTML markup on the page.
Complex React components:
- can include advanced logic that defines what the returned html will look like
- can contain it’s own state (more on state later)
- can contain lifecycle methods (more on that later)
- can include custom methods that will be executed when a user clicks on a button for example
That’s all you need to know. Now we’ll have a look at the syntax of both simple and more complex React components.
2. React component syntax
If you are coming from a jQuery background like me, you might find it overwhelming to look at the React class components, so lets break it down a little bit.
Simple (stateless) React components
01
02
03
04
| // Simple (stateless) React component const Headline = () => { return <h1>React Cheat Sheet</h1> } |
The simple React component is a function that returns HTML. In the above example
H1
will be rendered on a page.
You can also return multiple lines of HTML like this:
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
19
20
21
22
23
| // Component must only return ONE element (eg. DIV) const Intro = () => { return <div> <Headline /> <p>Welcome to the React world!</p> </div> } const Intro = () => { return ( <div> <Headline /> <p>Welcome to the React world!</p> </div> ) } const Intro = () => ( <div> <Headline /> <p>Welcome to the React world!</p> </div> ) |
See how we have included the parenthesis around the second example? (lines 10 and 15)
All three of these components would do the same thing, but including the parenthesis might help with readability of your components. Your choice.
The third example does not even include the return statement, but we had to change
{}
to ()
.
Why do we need the containing
div
around the HTML markup?
It is one of the requirements of JSX, each component can only return one element.
That is why you will need wrap all your HTML markup into one containing HTML element eg.
div
or ul
.
JSX makes writing your html inside of React components more elegant.
I will refer to JSX as HTML for the rest of this guide.
Advanced React components (ES6 classes)
Below is an example of React Class Component, sometimes referred to as a smart component.
01
02
03
04
05
06
07
| class App extends React.Component { render() { return ( <h1>React Cheat Sheet</h1> ) } } |
It does not make sense to use class for a simple component like that, you should use the stateless component instead.
Lets add a constructor, set current date as the local state of this component and then render it out inside of the
H1
.
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
19
20
21
22
23
| class App extends React.Component { // fires before component is mounted constructor(props) { // makes this refer to this component super (props); // set local state this .state = { date: new Date() }; } render() { return ( <h1> It is { this .state.date.toLocaleTimeString()}. </h1> ) } } |
Here we are Adding Local State to a Class.
Firstly we include the constructor with super() and then set the initial stateof the
App
inside of the this.state
JavaScript object.
We can now access the
date
from the state inside of the render method like this: {this.state.date}
.
2 important things to know about the React component’s state:
- you can not modify the state directly, you will need to use
this.setState({data: new Date()})
instead ofthis.state.date = new Date()
- state changes might be assynchronous, you should not rely on the state values for calculating the next state
Your state might grow with your app and include as many properties as you need.
Now lets see what the difference is between
state
and props
.3. State vs Props
You can pass some of your state values down to the child components as
props
.
Here is an example of a component that receives
props
:
01
02
03
04
| // Component that receives props const Greetings = (props) => { return <p>You will love it {props.name}.</p> } |
This component will render the
prop
name (Petr) if it was passed down from the parent component like this:
01
| <Greetings name={Petr}> |
We can then access this prop inside of the return statement as
{props.name}
or destructure the props right when we are creating this component like this:
01
02
03
| const Greetings = ({name}) => { return <p>You will love it {name}.</p> } |
This will let us only use
{name}
inside of the return statement.
The main difference between props and state in React is that
props
are read-only and can not be modified from inside of component.
If we wanted to change the
name
rendered inside of Greetings
component we would need to go the parent React component, modify the name and pass down the new value.4. Destructuring
I mentioned the word destructure, it refers to the concept of ES6 destruring.
Instead of creating multiple variables (constants) like this:
01
02
03
04
| const name = this .props.name; const age = this .props.age; const isLoggedIn = this .state.isLoggedIn; const username = this .state.username; |
Get used to destructuring like this:
01
02
| const {name, age} = this .props; const {isLoggedIn, username} = this .state; |
It will save you some typing and make your code more compact.
Great new ES6 feature build into JavaScript.
5. Render component into the DOM
To be able to see your React component on the page you will need to use
ReactDOM.render()
and pass it an html element where you want your app to be mounted to.
01
02
03
04
05
06
07
08
09
10
11
12
| // your-project-folder/src/index.js // Import React and ReactDOM import React from 'react' import ReactDOM from 'react-dom' ; import App from './js/components/App' ; // Render component into the DOM - only once per app ReactDOM.render( <App />, document.getElementById( 'root' ) ); |
In the above example we are firstly importing React and ReactDOM
Then we are importing the
App
component from another file and mounting it to the page into the div#root
element.
You will find this
ReactDOM.render()
method inside of every React app.
This code is inside of a file called “entry point” and mostly called
index.js
or similar.Conclusion and your challenge
Now you are familiar with the basic React concepts such as components, state, props, destructuring and mounting.
Your challenge
Explore the following CodePen demo and see if you can answers some of these questions.
- Can you see the entry point and the related “mounting” code?
- How many React components are in this
app
? - Which line of the code is using
destructuring
? - Which of the two React components does not have to be a class component and why?
- Can you rewrite that component into a stateless component?
Give it a try.
Have you found answering these questions much easier then before reading this article? Let me know in the comments.
We will keep exploring the other parts of React in the next article.
Do you want to learn even more about React?
- Download and explore React Cheat Sheet PDF.
- Check out my React Tutorials 2017 playlist.
- Take my free React online course and build 3 practical React components from scratch.
test
ReplyDelete